Overview
AlgoBase is primarily a problem management application, where users can add different algorithm problems from all kinds of sources. Within the application, users can create training plans consisting of tasks, where each task is a problem that the user has to complete. Upon completing a task, users can mark it as done, which contributes to the progress of their plan. Users interact with AlgoBase using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 20 kLoC.
The figure below illustrates the layout of the AlgoBase application:
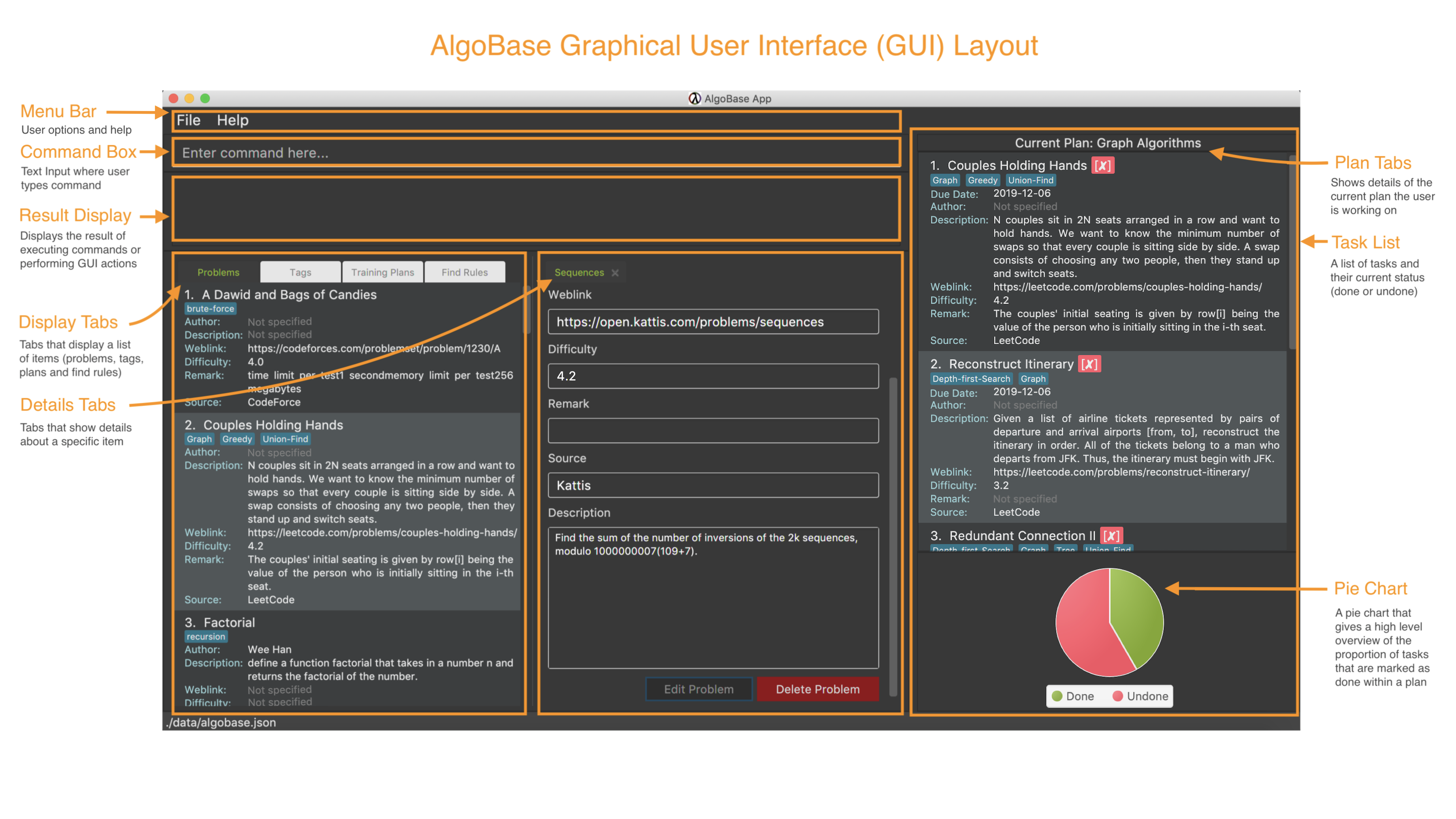
My role was to design and write the codes for the training plan feature. The following sections illustrate these enhancements in more detail, as well as the relevant documentation I have added to the user guide and developer guide.
Summary of contributions
-
Major enhancement: added the training plan feature
-
What it does: Allows users to create customized training plans with specific starting date and end date and that consist of selected problems in AlgoBase. Each problem is wrapped up as a task in the plan. Users can record their progress by marking problems in plans as done or undone, and they can edit, delete or search for plans.
-
Justification: This feature improves the product significantly because it would be inconvenient for a hard-working user to manage a large number of problems without a higher-level component that categorize selected problems.
-
Highlights: This feature enables users to set specific duration for a training plan, which provides the possibility of various potential enhancements in the future, such as alarming for deadlines, and thus will make AlgoBase more attractive to users.
-
-
Minor enhancement to existing project: refactored part of command and parsers
-
Code contributed: Code contributed
-
Other contributions:
-
Project management:
-
Managed release
v1.3
on GitHub
-
-
Enhancements to project prototype:
-
Community:
-
Reported bugs and suggestions for other teams in the class: PE Dry Run
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Training Plans
Training plans consist of wrapped-up problems which we call task
s.
Adding a plan: addplan
Generates a new plan.
Format: addplan n/NAME [d/DESCRIPTION] [start/START_DATE] [end/END_DATE]
-
Plans cannot have duplicate names.
-
START_DATE
andEND_DATE
should be in the format ofyyyy-MM-dd
-
START_DATE
should be before or equal toEND_DATE
. -
START_DATE
will be set toLocalDate#now()
if not specified, andEND_DATE
will be one month fromSTART_DATE
if not specified.
Examples:
-
addplan n/CS2040 d/past year questions in practical exams start/2019-01-01 end/3019-12-12
Deleting a plan : deleteplan
Deletes an existing plan.
Format: deleteplan INDEX
-
Deletes the plan at the specified
INDEX
. -
The index refers to the index number shown in the displayed plan list.
-
The index must be a positive integer 1, 2, 3, …
Examples:
-
listplan
deleteplan 2
Deletes the second plan in the displayed list. -
findplan n/CS2040
delete 1
Deletes the first plan in the results of thefindplan
command (will be introduced in the next section).
Editing a plan : editplan
Edits an existing plan.
Format: editplan INDEX [n/NAME] [d/DESCRIPTION] [start/START_DATE] [end/END_DATE] [f/]
-
Edits the plan at the specified
INDEX
. The index refers to the index number shown in the displayed plan list. The index must be a positive integer 1, 2, 3, … -
At least one of the optional fields must be provided.
-
Existing values will be updated to the input values.
-
START_DATE
should be before or equal toEND_DATE
. -
The time range of the edited plan should cover due dates of its tasks. However, adding
f/
will force inconsistent due dates to change to the plan’s end date.
Examples:
-
editplan 1 d/past year questions of sit-in labs
Edits the description of the first plan to bepast year questions of sit-in labs
respectively. -
editplan 2 start/2019-08-13 f/
Edits the starting date of the second plan to be2019-08-13
and any task with due date before2019-08-13
to the plan’s end date.
Listing all plans : listplan
Displays a list of all existing plans.
Format: listplan
Locating plans: findplan
Finds plans fulfilling all provided constraints.
Format: findplan [n/NAME] [d/DESCRIPTION] [start/RANGE_START] [end/RANGE_END] [task/TASK_NAME]
-
Name
-
is case-insensitive.
-
is considered a match as long as one word matches.
-
is matched word by word.
-
-
Description
-
is case-insensitive
-
is matched word by word
-
is considered as a match only when the problem’s description includes all words in the constraint.
-
-
Start and end range
-
is considered a match when there exist overlaps in time. (e.g. a plan with start date of
2019-01-01
and end date of2019-03-03
matches a plan with start date of2019-02-02
and end date of2019-04-04
) -
both
RANGE_START
andRANGE_END
should be specified. -
RANGE_START
should be before or equal toRANGE_END
.
-
-
Task name
-
is case-sensitive.
-
is matched word by word.
-
is considered a match only when there is a task whose name is exactly the same as the given task name.
-
Examples:
-
findplan start/2019-08-05 end/2019-12-07
Finds plans whose duration overlaps with NUS AY 19/20 Semester 1. -
findplan task/Sequences
Finds plans that contain a task named 'Sequences'.
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Training Plan Feature
Training plan feature allows users to create customized training plans with specific starting date and end date and that consist of selected problems in AlgoBase. Each problem is wrapped up as a task in the plan. Users can record their progress by marking problems in plans as done or undone, and they can edit, delete or search for plans.
Current Implementation
The training plan mechanism is faciliated by AlgoBase
, which keeps a list of training plans. It supports the following operations:
-
Algobase#addPlan()
— Adds a new training plan. -
AlgoBase#setPlan()
— Replaces an existing plan by an edited version. -
AlgoBase#removePlan()
— Deletes a training plan. -
AlgoBase#getPlanList()
— Returns a list of training plans.
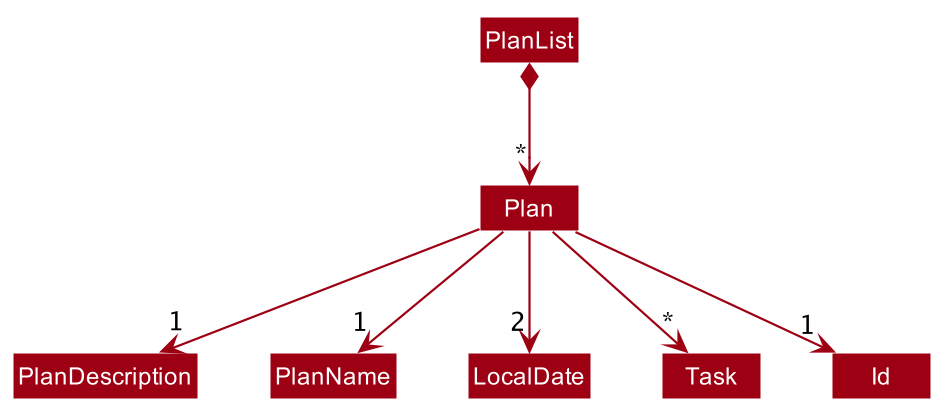
Plan
Given below is an example usage scenario and how the AlgoBase behaves at each step.
Step 1. The user launches the application for the first time. The AlgoBase
will be initialized with the initial empty state.
Step 2. The user switches to the plan tab and executes addplan n/CS2040 d/past year questions start/2019-01-01 end/2019-05-04
command to add a new plan to AlgoBase. The addplan
command checks if Model#hasPlan()
and calls Model#addPlan()
, causing the modified state of plans after the addplan
command executes to be saved in the PlanList
.
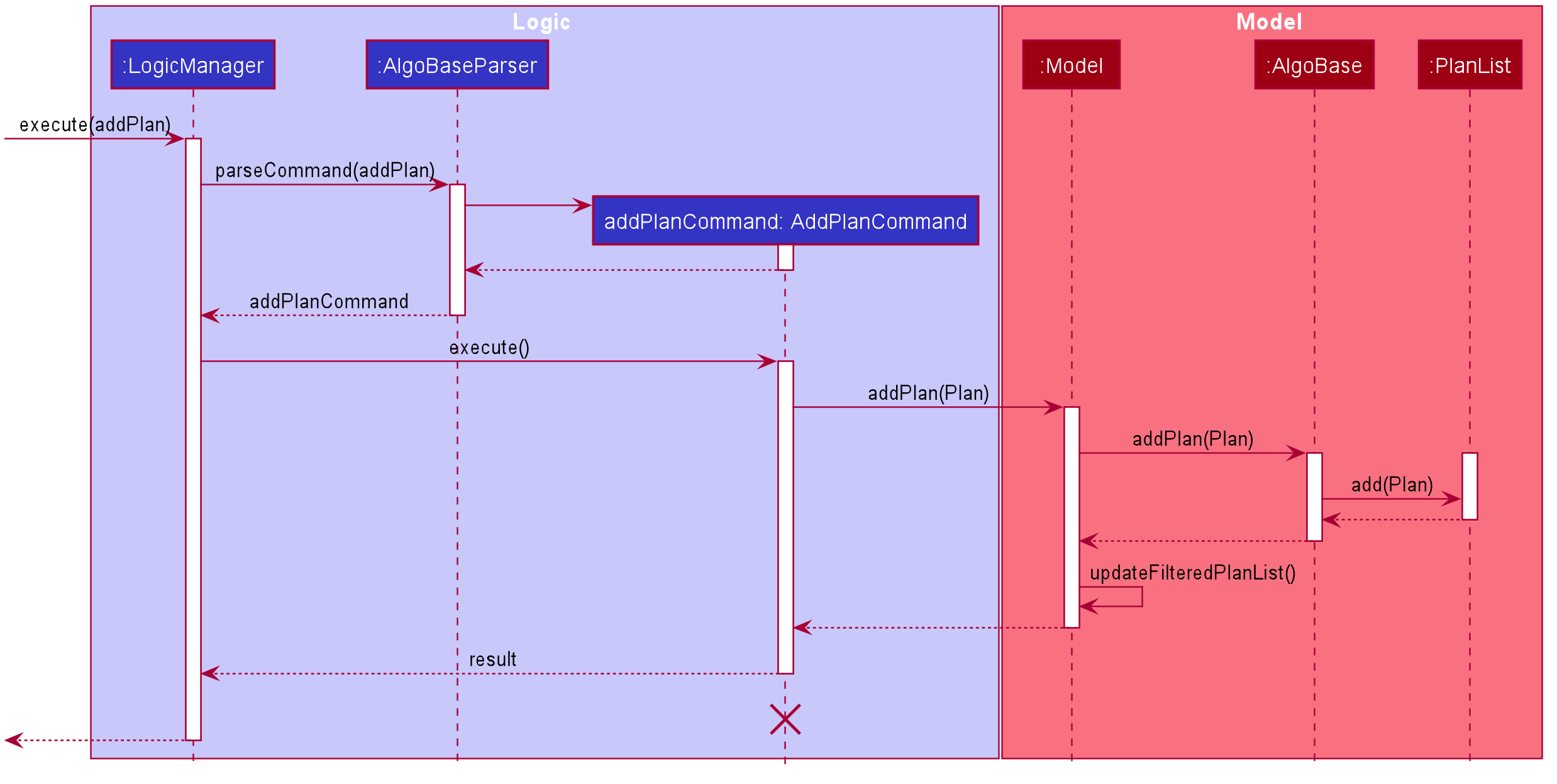
AddPlanCommand
Step 3. The user executes listplan
to list all plans. The listplan
command calls Model#updateFilteredPlanList()
. The plan CS2040
is numbered 1
in the displayed list.
Step 4. The user finds out that the exam date of CS2040 has changed, and decides to change the end date of the training plan by executing the editplan 1 end/2019-05-05
command. The editplan
command will check if Model#hasPlan()
, and then call Model#setPlan()
and Model#updateFilteredPlanList()
, which will replace the original plan with the modified plan in the PlanList
.
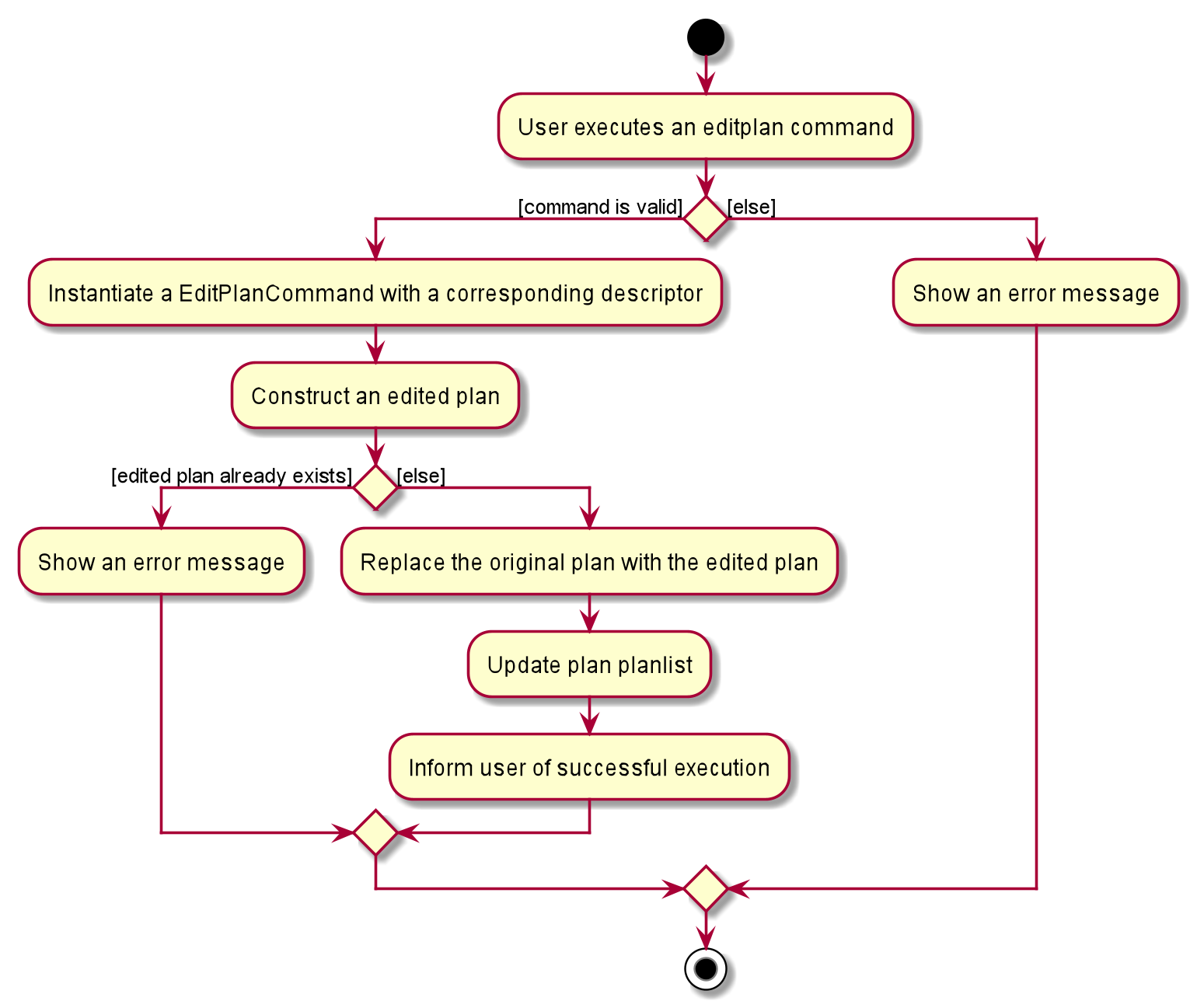
EditPlanCommand
The user can also add value for an empty field by executing editplan command if the field has not been specified when adding the plan.
|
Step 5. The user then decides to execute the command findplan start/2019-03-01 end/2019-03-31
to find out what plans he has in March. The findplan
command constructs a FindPlanDescriptor
, and then executes Model#getFilteredPlanList()
and Model#updateFilteredPlanList(FindPlanDescriptor)
. A list of plans in AlgoBase that has overlapping time range with the specified starting date and end date will be displayed on the plan list panel.
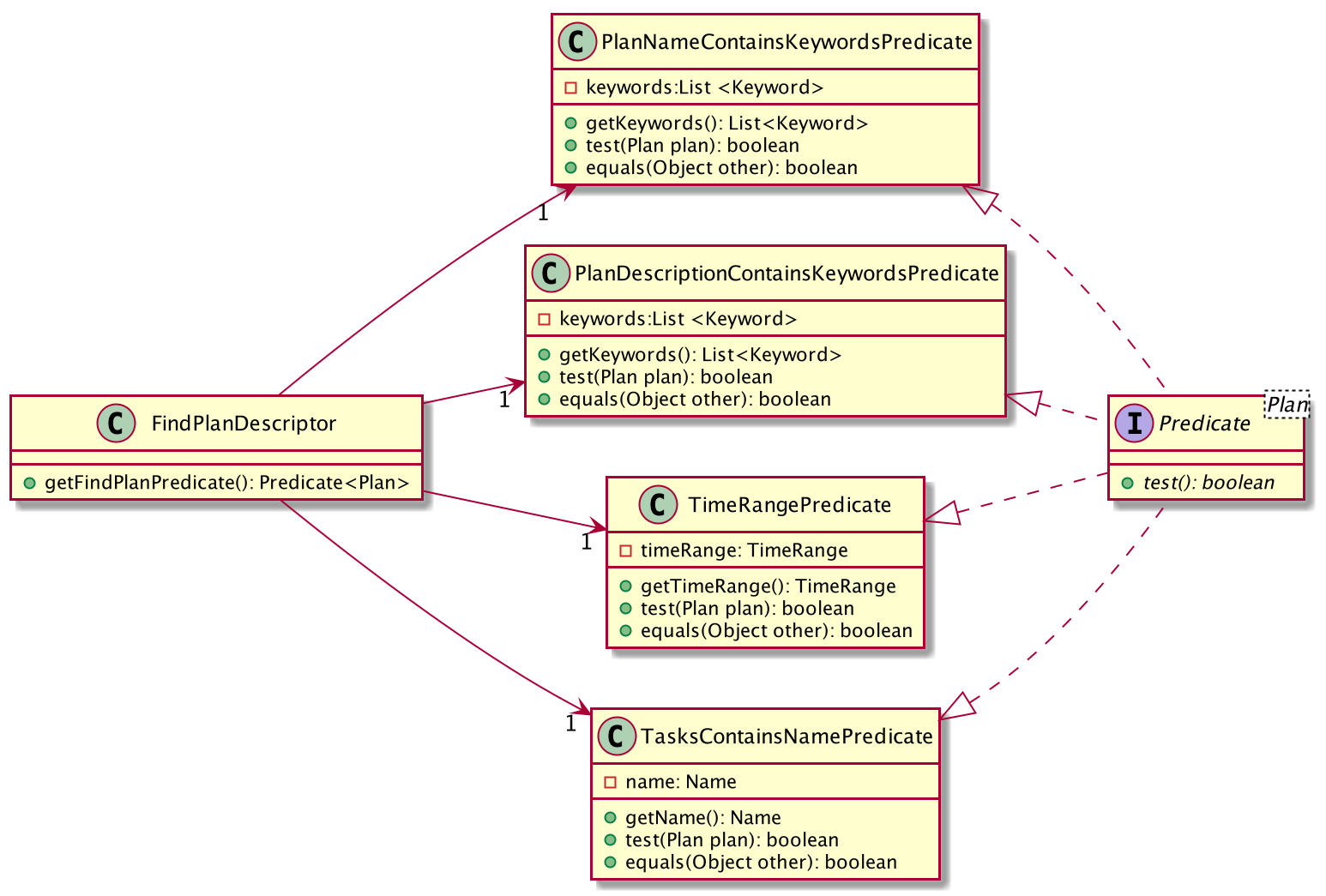
FindPlanDescriptor
If the user wants to find plans with overlapping time range, both the starting date and the end date should be specified, and the starting date should be before or at least equal to the end date, or an error message will be displayed to inform the user the correct form of input. |
Step 6. The user executes deleteplan 1
, which calls Model#getFilteredPlanList()
and Model#deletePlan
. The Model#getFilteredPlanList()
returns the last shown plan list, which is list of plans returns by the findplan
command in step 4. Therefore, the first plan with overlapping time range is deleted.
Design Considerations
Aspect: Data Structure of TimeRange class
-
Alternative 1 (current choice): Abstract out a
TimeRange
class in packageplansearchrule
.-
Pros: Easy to implement.
-
Cons: Generating a
TimeRange
object infindplan
command adds coupling, and is not very intuitive.
-
-
Alternative 2: Abstract out
startDate
andendDate
fields in plans to a single fieldTimeRange
.-
Pros: More OOP (
startDate
andendDate
are currentlyLocalDate
objects). -
Cons: We must ensure that the implementation complies with other date-related commands and storage of plans, such as adding or editing due dates of tasks in plans and the json file.
-
Aspect: How to find plans with certain tasks
-
Alternative 1 (current choice): By exactly-matching names.
-
Pros: Easy to implement.
-
Cons: Users need to figure out the exact name of the task they would like to find, which is more time-consuming.
-
-
Alternative 2: By indicating index of the original problem.
-
Pros: Complies with other usages of prefix
task/
. -
Cons: Adds coupling due to access to
filteredProblemList
in the model.
-
-
Alternative 3: By exactly-matching tags of the original problem.
-
Pros: User-friendly.
-
Cons: Adds coupling due to access to
filteredProblemList
in the model as the wrapped-up task does not have a tag list field.
-
Plan
Adding a plan
-
Test case:
addplan n/ByteDance d/coding test for Software engineering
Expected: A new plan with respective information is added and displayed on plan tab. Start date is now and end date is one month from now. -
Test case:
addplan n/ByteDance d/coding test for Software engineering
Expected: No plan is added. No plans with duplicate names can be added. Error details shown in the status message. -
Test case:
addplan n/Graph start/2019-1-1 end/2019-3-3
Expected: No plan is added. The date should be inyyyy-MM-dd
format. Error details shown in the status message. -
Test case:
addplan n/Graph end/1912-06-23
Expected: No plan is added. If no starting time is specified, the starting time will beLocalDate#now()
, and the starting time should not be after end time. Error details shown in the status message.
Editing a plan
-
Prerequisites: List all plans using the
listplan
orfindplan
command. Multiple plans in the list. -
Test case:
editplan 1 d/give up start/2019-01-01 end/2020-02-02
Expected: The description and the dates of the first displayed plan is changed. -
Test case:
editplan 1 end/2018-02-02
Expected: Edit command is invalid. Error details shown in the status message. Starting date should be before or equal to end date. -
Test case:
editplan 1 end/2019-02-02 f/
Expected: The end date of the first displayed plan is changed to2019-02-02
. Any task’s due date after2019-02-02
will be changed to2019-02-02
. ..Test case:editplan 1 start/2019-01-30 f/
Expected: The starting date of the first displayed plan is changed to2019-01-30
. Any task’s due date before2019-01-30
will be changed to the plan’s end date.
Finding plans
-
Test case:
findplan n/bytedance
Expected: The plan named 'ByteDance' is listed. -
Test case:
findplan start/2019-01-01 end/2019-12-12
Expected: Plans whose time range overlaps with the given time range are listed in the plan panel. -
Test case:
findplan start/2019-01-01
Expected: Invalid command. Both range start and range end should be specified. Error details shown in the status message. -
Test case:
findplan start/2019-01-01 end/2018-01-02
Expected: Invalid command. Range start should not be after range end. Error details shown in the status message.
Deleting a plan
-
Prerequisites: List all plans using the
listplan
orfindplan
command. Multiple plans in the list. -
Test case:
findplan n/bytedance
deleteplan 1
Expected: The plan with name 'ByteDance' is deleted. -
Test case:
listplan
deleteplan 1
Expected: The first plan among all plans is deleted.
Listing plans
-
Test case:
listplan
Expected: All plans in Algobase are listed in the plan display tab.