Overview
AlgoBase is a desktop algorithmic problem manager. The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 20 kLoC.
Summary of contributions
-
Code contributed: Code contributed
-
Major enhancement: Implemented features for the Graphical User Interface
-
What it does: allows the user to open problems & plans in their own separate tabs, where they can edit and delete said items in the GUI in a user-friendly manner.
-
Justifications: As our target users are Computer Science students preparing for technical interviews, they would likely want to manage multiple plans and problems concurrently. We decided to introduce tabbing to address this need, as it is a convenient, intuitive and user-friendly solution. Furthermore, while the command line is fast for typing short commands, it might not be ideal in certain cases. For example, it would be extremely tedious and time consuming to edit large amounts of text from the command line. Hence, I implemented the ability to edit a problem or plan from within the tabs. Not having to deal with cumbersome details tremendously improves the overall user experience our user – it reduces their frustration and maximises the time they spend practicing for their interviews
-
Highlights:
-
This enhancement introduces a new high level component
UiLogic
, which handle events, orUiActions
, triggered by the user in the GUI. It was challenging as logic of the Command line is primarily synchronous while the GUI is event-driven. Integrating the 2 architectural styles cleanly required an in-depth evaluation of design alternatives. -
Furthermore, this feature transcends across all 5 components of AlgoBase:
UI
,Logic
,UiLogic
,Model
andStorage
, making it a full-stack development consisting of more than 4000 lines of functional code and more than 1500 lines of tests.
-
-
-
Minor enhancement: Command Line Tab management.
-
What it does: allows the user to manage (i.e. switch, open and close) tabs from the Command Line.
-
Justification: While a GUI would make sense for tedious actions like editing, the command line is arguably faster for simpler actions like switching tabs. Furthermore, our target users are Computer Science students who are used to command line window / tab management (through vim and emacs). Hence, I decided to implement a command line version for tab management to optimize for these users, which will help improve their user experience.
-
-
Other contributions:
-
Project management:
-
Managed releases
v1.2
-v1.2.1
(2 releases) on GitHub
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Tools:
-
Integrated a new Github plugin (Travis-CI) to the team repo
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Overview
AlgoBase is primarily a problem management application, where users can add different algorithm problems from all kinds of sources. Within the application, users can create training plans consisting of tasks, where each task is a problem that the user has to complete. Upon completing a task, users can mark it as done, which contributes to the progress of their plan.
The figure below illustrates the layout of the AlgoBase application:
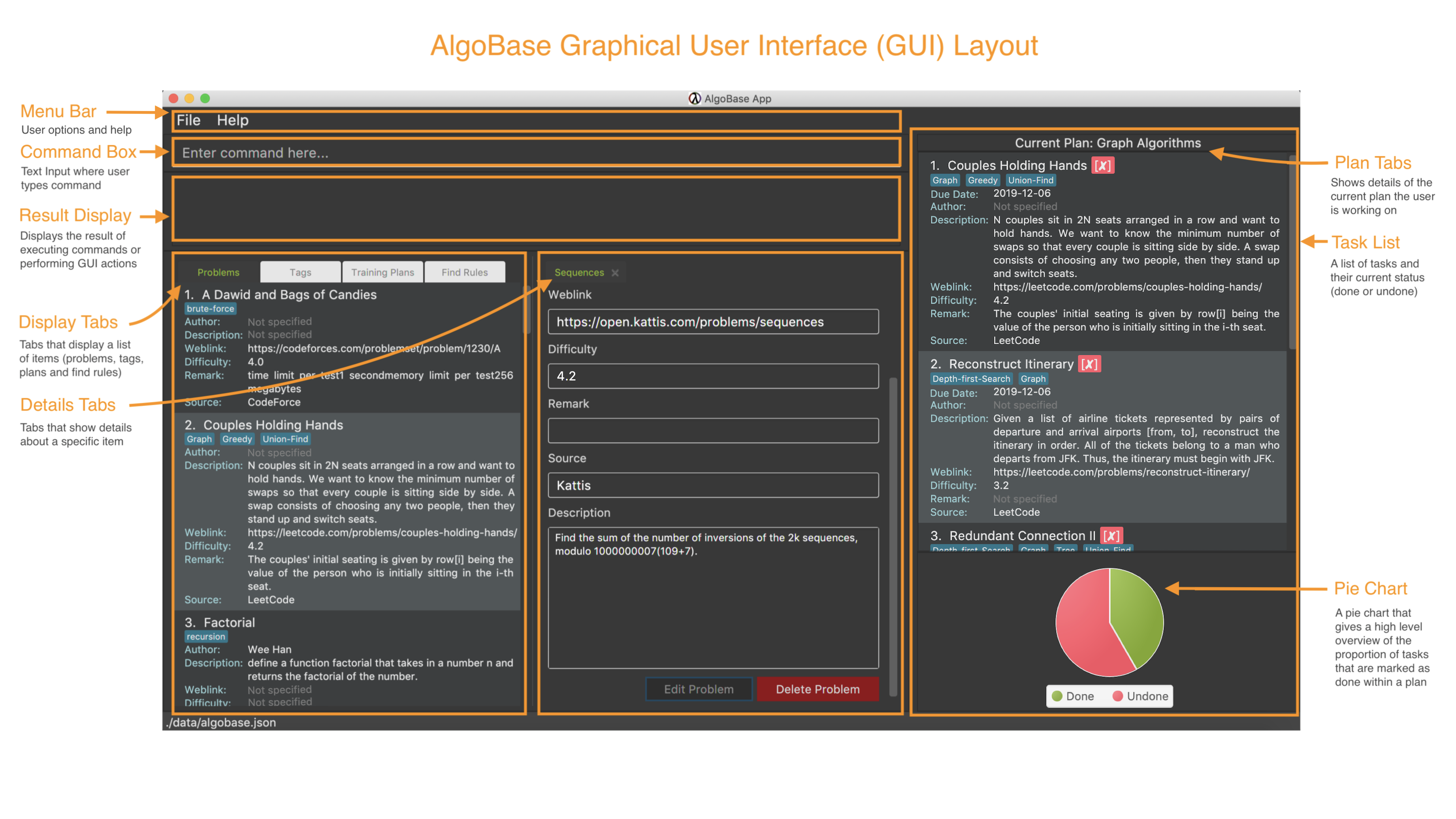
AlgoBase is split into following 3 panes:
-
Display Tabs – displays a list of items (problems, tags, plans or find rules)
-
Details Tabs – displays details of a specific item
-
Task Management Pane – displays details of the current plan === Graphical User Interface (GUI) Enhancements
AlgoBase currently supports several GUI features with an intuitive layout, including the following:
-
Opening, Closing and Switching between Tabs
-
Editing a Problem or Plan
-
Deleting a Problem or Plan
Managing Tabs
There are 3 types of tabs in AlgoBase, 2 of which (Display and Details) are shown in the figure below.
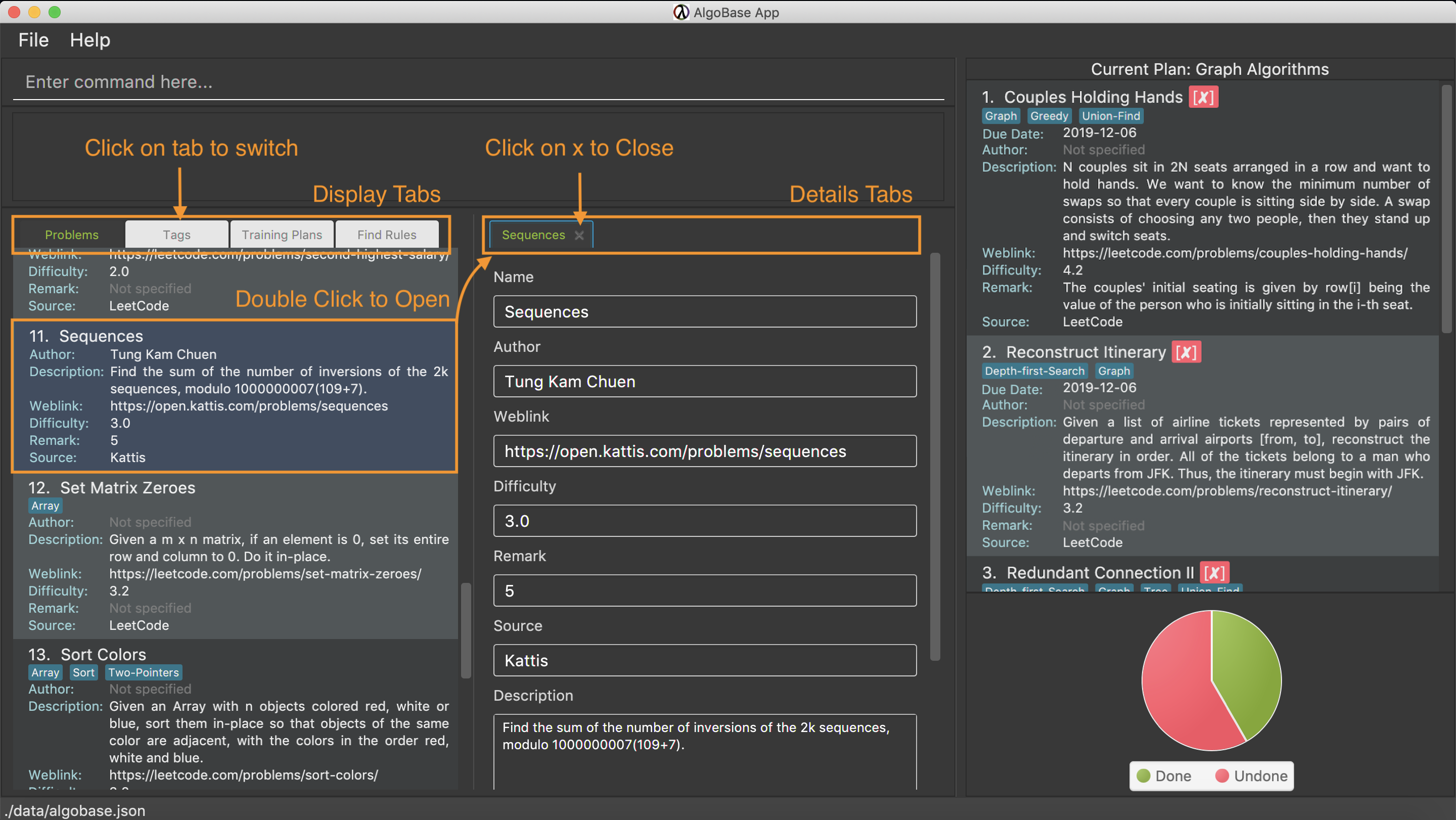
-
Display tabs give a high level overview of the contents of a list of items
-
Details tabs give a more detailed description of an item in a display tab.
Much like tabs you see in other applications, tabs in AlgoBase work the same way.
-
To Switch Tabs – Click on a tab to switch tabs
-
To Open a Tab – Double click on an item in the Display tab to open it in the Details tab
-
To Close a Tab – Click on the
x
button next to a Details tab to close it.
Display Tabs cannot be closed. |
Editing a Problem or Plan
The following steps show how a user can edit problems or plans from the GUI.
Step 1: Select the problem or plan you want to delete by double clicking on it. Step 2: Make changes to the problem or plan by editing the fields directly.
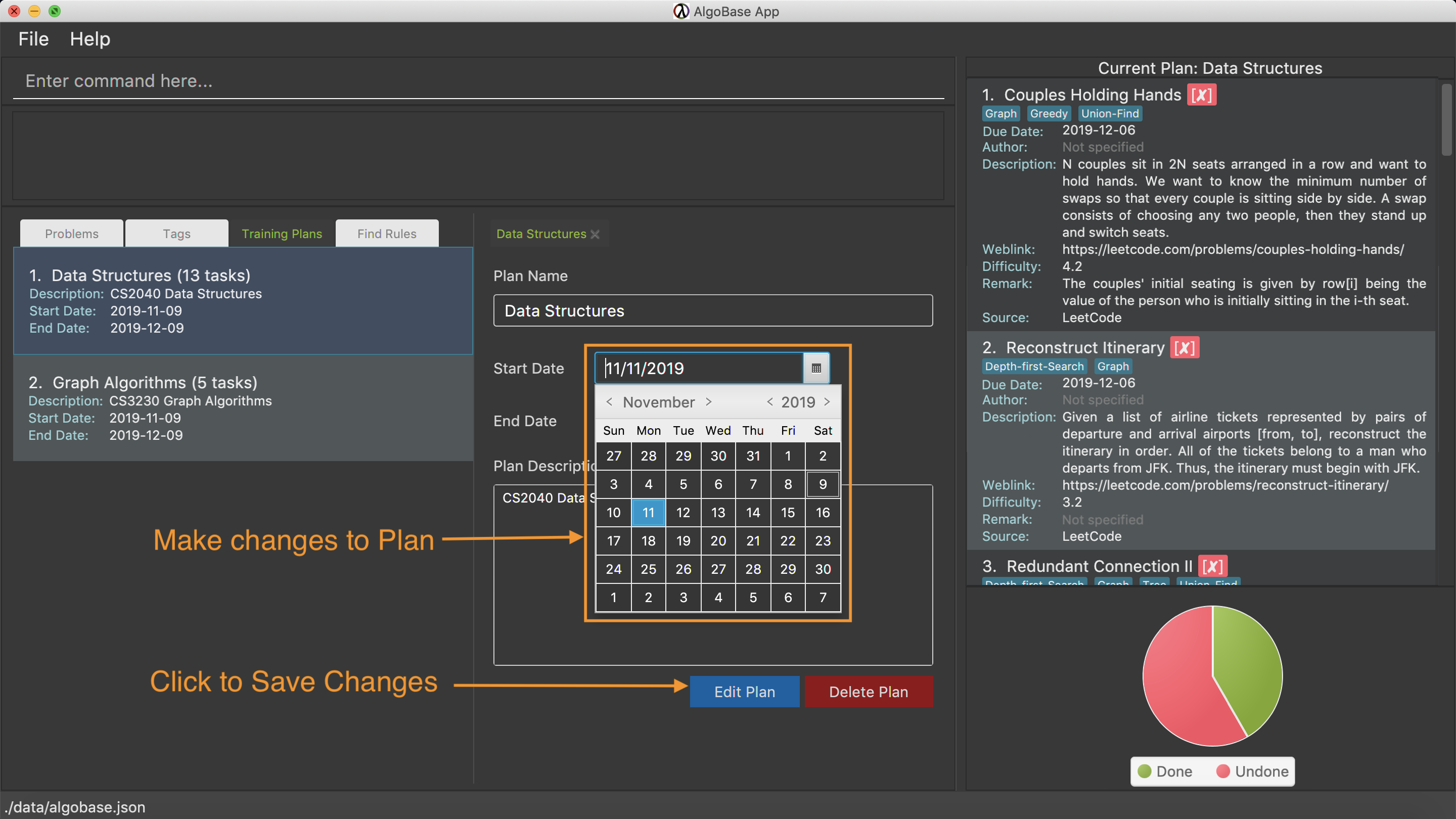
Step 3: Save changes to the problem or plan by clicking on the Edit Problem or Edit Plan button.
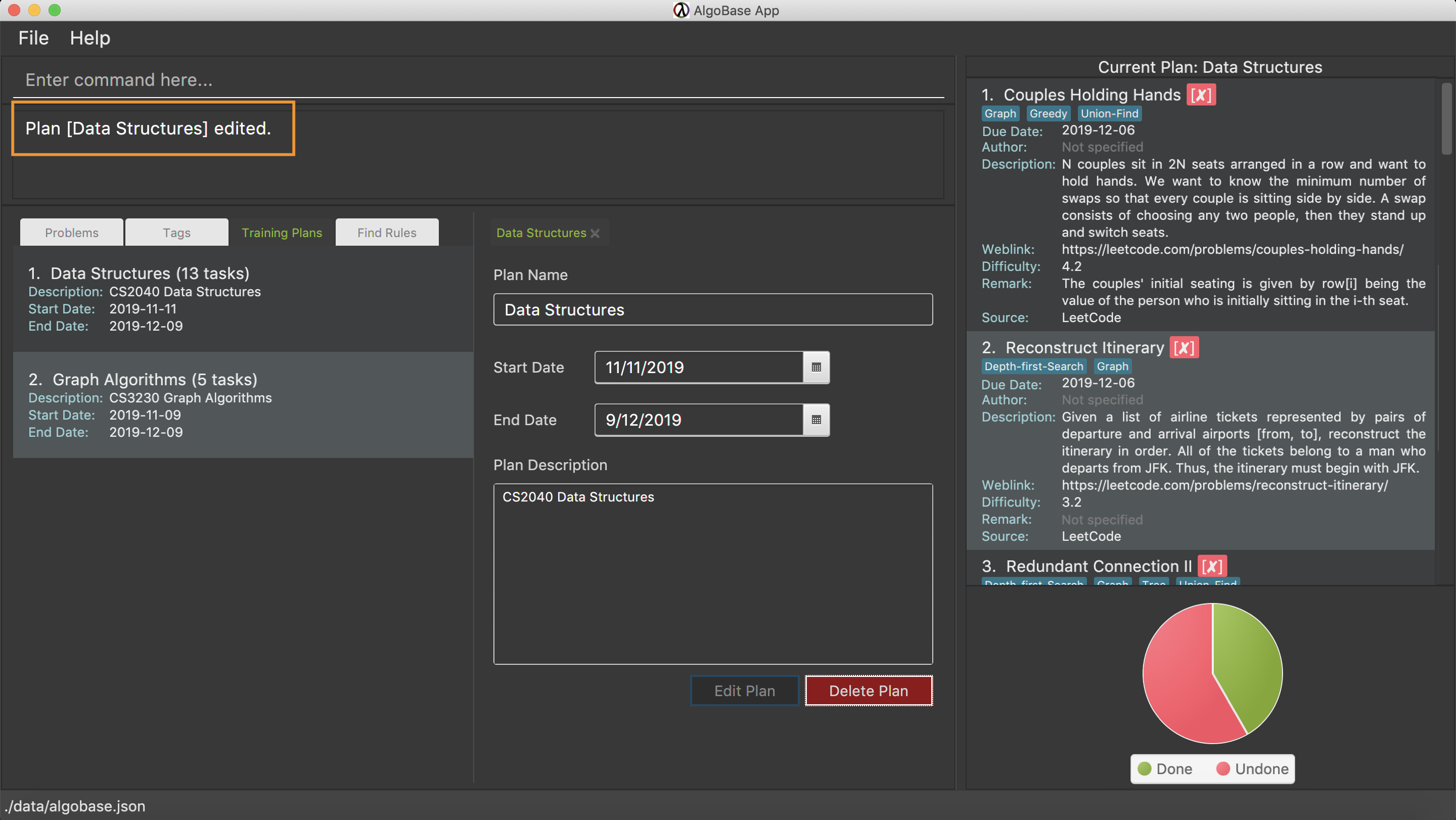
The Edit Plan Button cannot be clicked if there are no changes made to the plan. |
Deleting a Problem or Plan
The following steps show how a user can delete problems or plans from the GUI.
Step 1: Select the problem or plan you want to delete by double clicking on it. Step 2: Click on the red Delete Problem or Delete Plan button at the bottom right. To prevent accidental deletion, a warning dialog will appear to confirm if you would like to delete the item. Click on the "confirm" button.
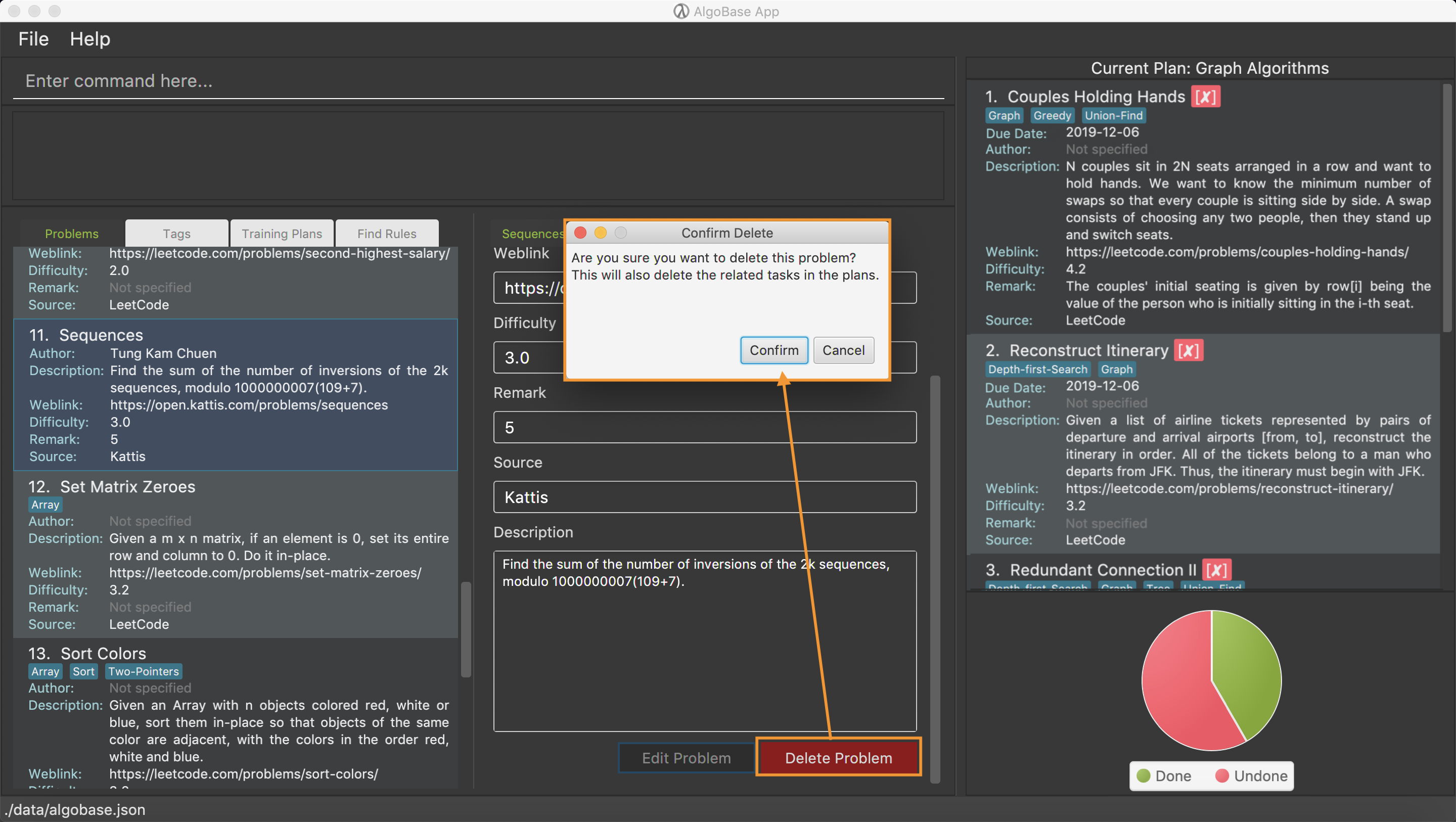
Step 3: Click on Confirm.
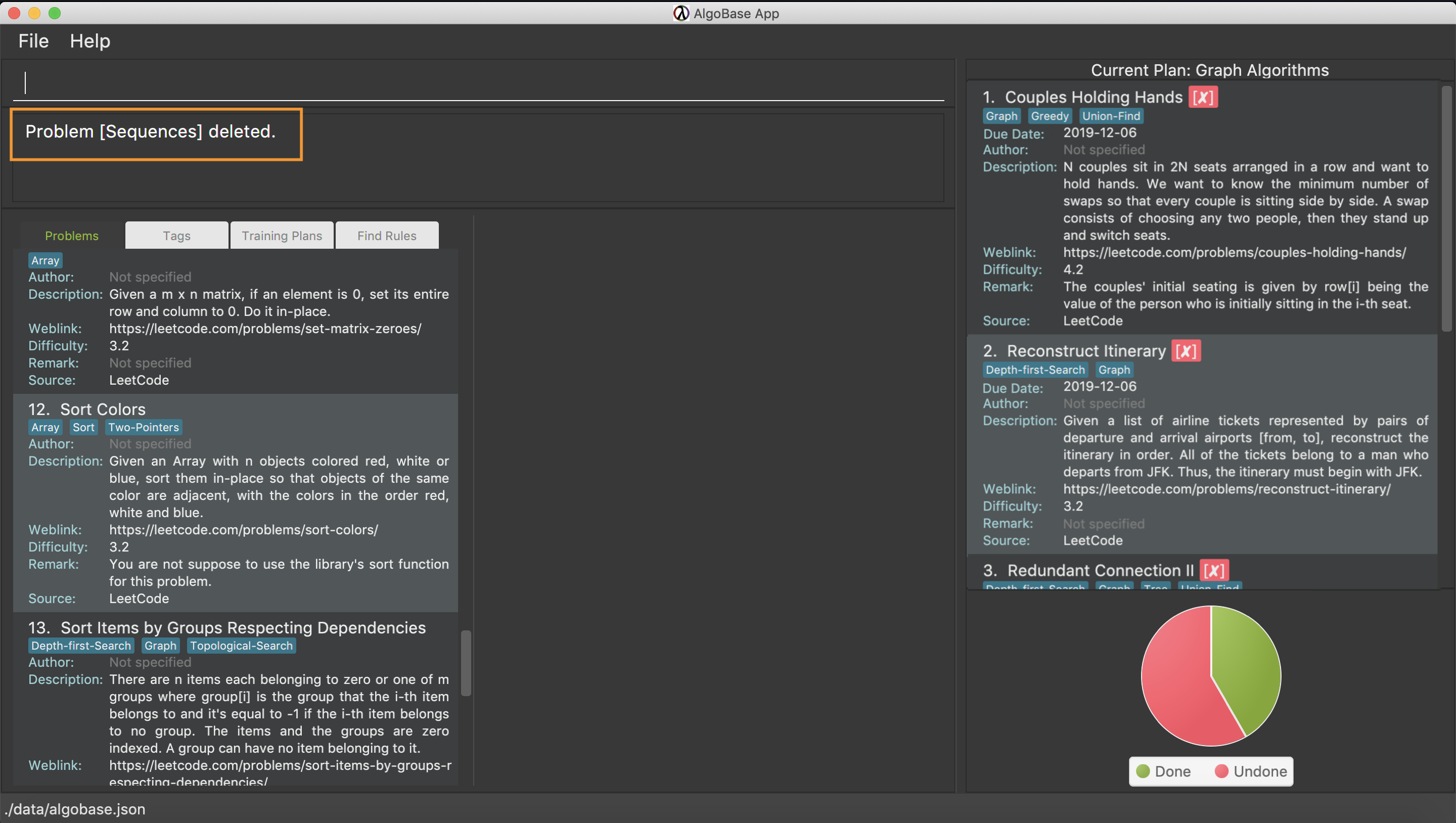
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
UiLogic Component
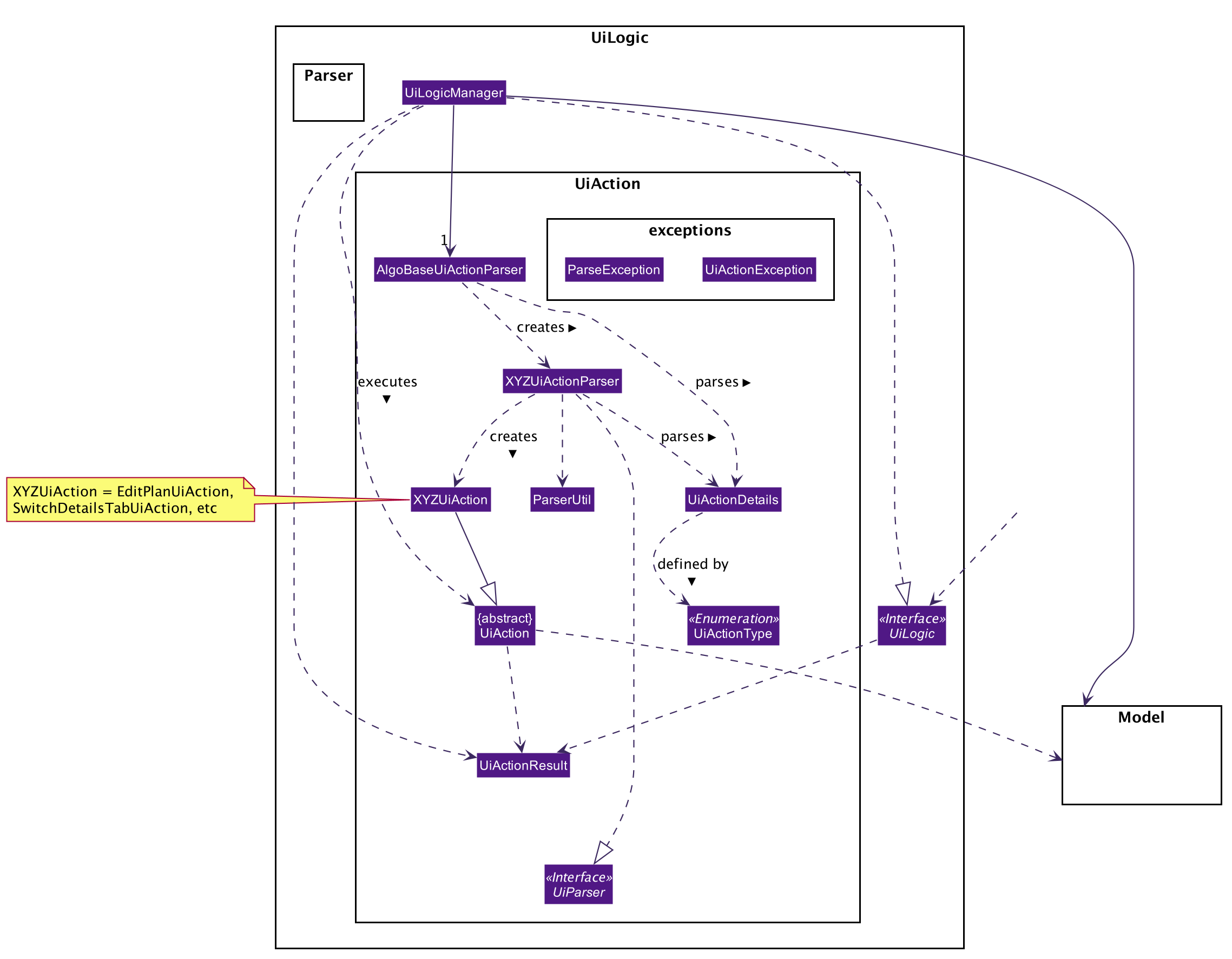
API :
UiLogic.java
-
Performing an action (e.g. switching tabs) triggers the creation of a
UiActionDetails
object. -
UiLogic
uses theAlgoBaseUiActionParser
class to parse theUiActionDetails
object. -
This results in a
UiAction
object which is executed by theUiLogicManager
. -
The command execution can affect the
Model
(e.g. deleting a problem). -
The result of the command execution is encapsulated as a
UiActionResult
object which is passed back to theUi
. -
In addition, the
UiActionResult
object can also instruct theUi
to perform certain actions, such as displaying the results as feedback to the user.
Graphical User Interface Enhancements
Current Implementation
The following classes facilitate the handling of GUI actions:
-
UiActionType
- An Enum of the types of UI actions that exist in AlgoBase. -
UiActionDetails
- An object containing details of a UI action. -
UiAction
- Interface with instructions for executing a UI action. -
UiLogicManager
- ImplementsUilogic
and manages the overall UI Logic. -
AlgoBaseUiActionParser
- Parses aUiActionDetails
object into an implementation ofUiAction
. -
UiActionResult
- The result of executing the UI action.
When the user makes a change in the GUI, the change is propagated from Ui
to UiLogic
to Model
and to Storage
, as represented in the diagram below:
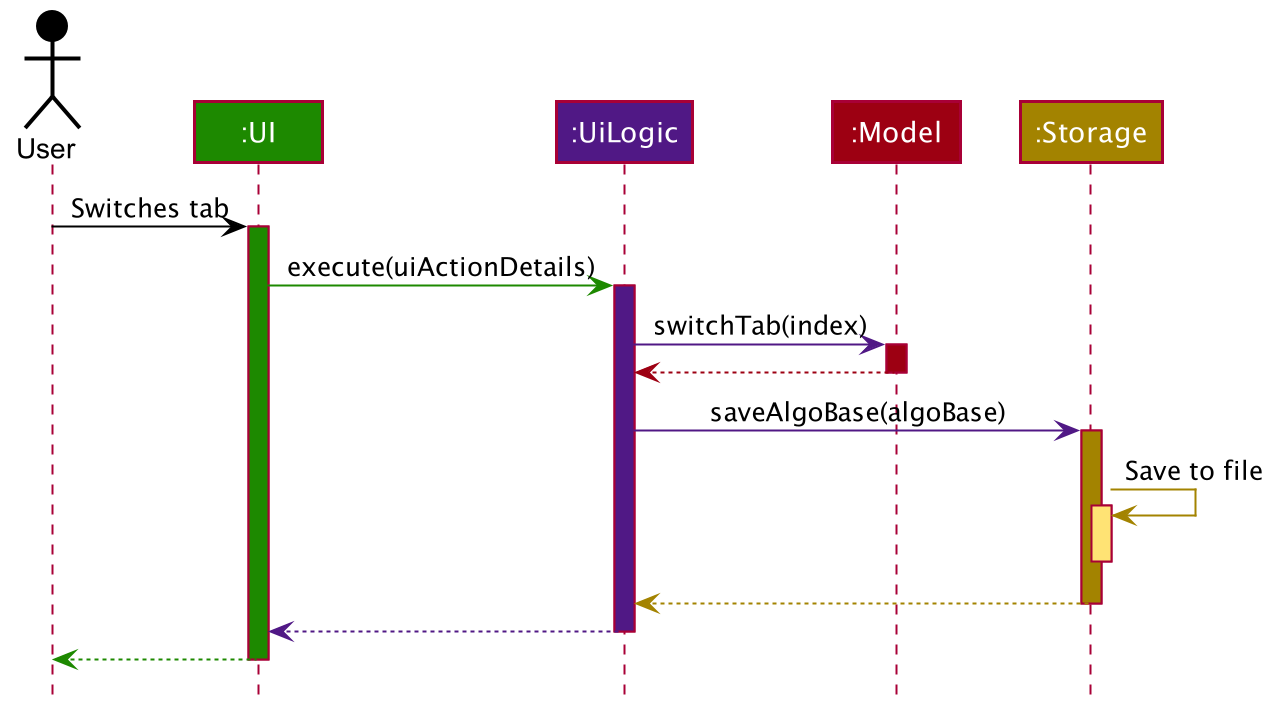
This process of how the application handles UI Actions is captured by the example in the Sequence Diagrams below:
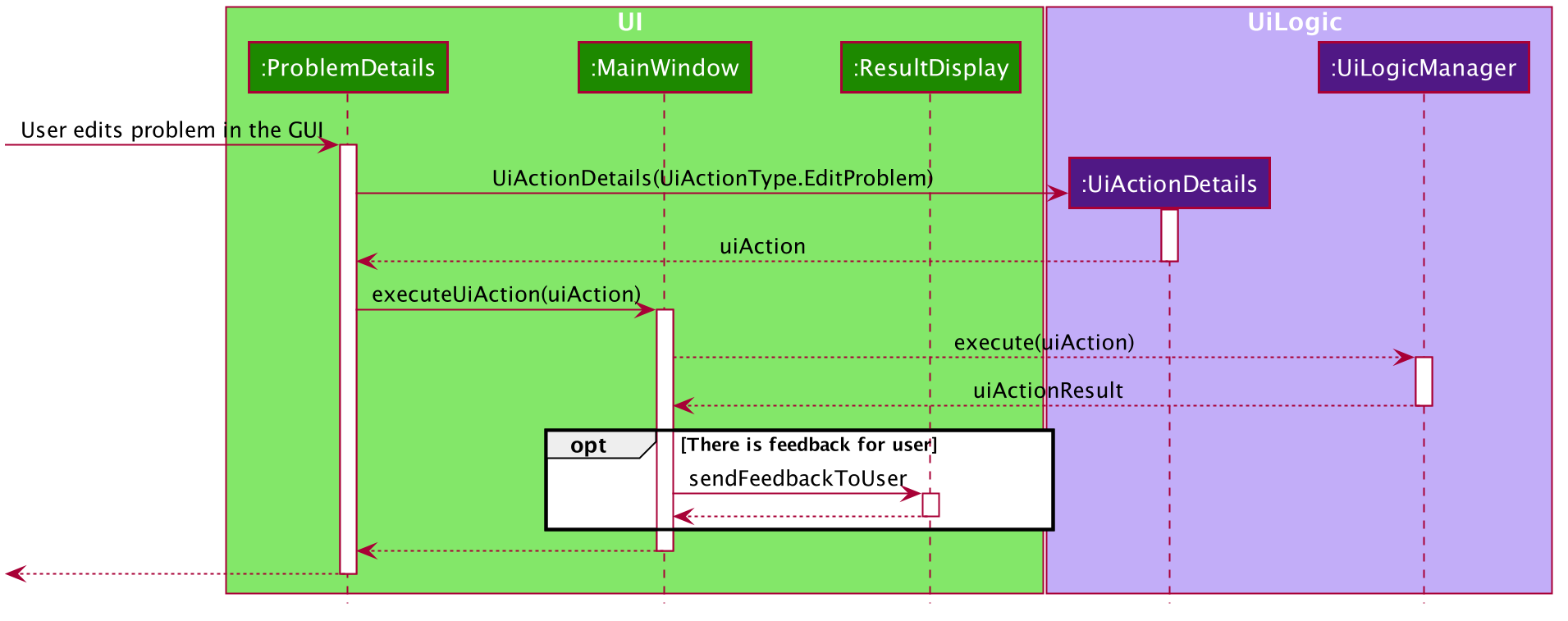
UI
and UiLogic
Step 1: The user edits the ProblemDetails
controller class through his/her actions in the GUI.
Step 2: The ProblemDetails
class constructs a new UiActionDetails
object of type UiActionType.EditProblem
.
Step 3: The executeUiAction
of the MainWindow
class is called with the UiActionDetails
object,
which in turn calls the execute
method of UiLogicManager
.
Step 4: The method call returns a UiActionResult
object, which may optionally contain feedback for the user.
The following diagram goes into more details on how the UiLogic
handles the UiActionDetails
:
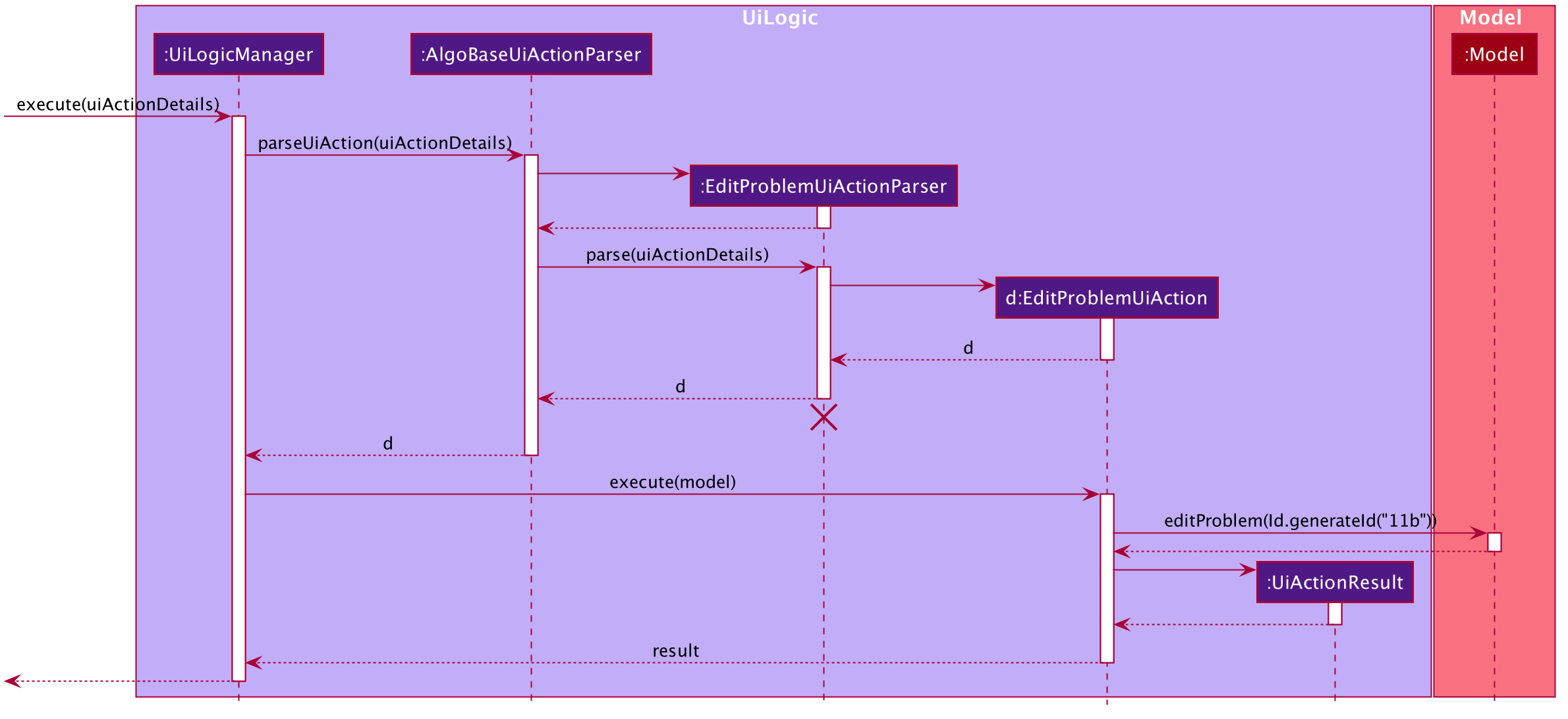
UiLogic
component.Step 1: The UiLogicManager
passes the UiActionDetails
object to the AlgoBaseUiActionParser
,
which in turn passes it to the EditProblemUiActionParser
based on its Action type.
Step 2: The EditProblemUiActionParser
converts the UiActionDetails
object into a EditProblemUiAction
object,
and passes it back to the UiLogicManager
.
Step 3: The UiLogicManager
executes the EditProblemUiAction
together with the Model
, and returns the UiActionResult
.
Graphical User Interface State
Current Implementation
The state of the GUI is stored in a GuiState
object, which is in turn stored in the Model
. The GuiState
object contains a TabManager
object, which manages tab information such as the tabs that are open and the tabs that are currently selected.
The following class diagram illustrates how the classes in the GuiState
interact with one another:
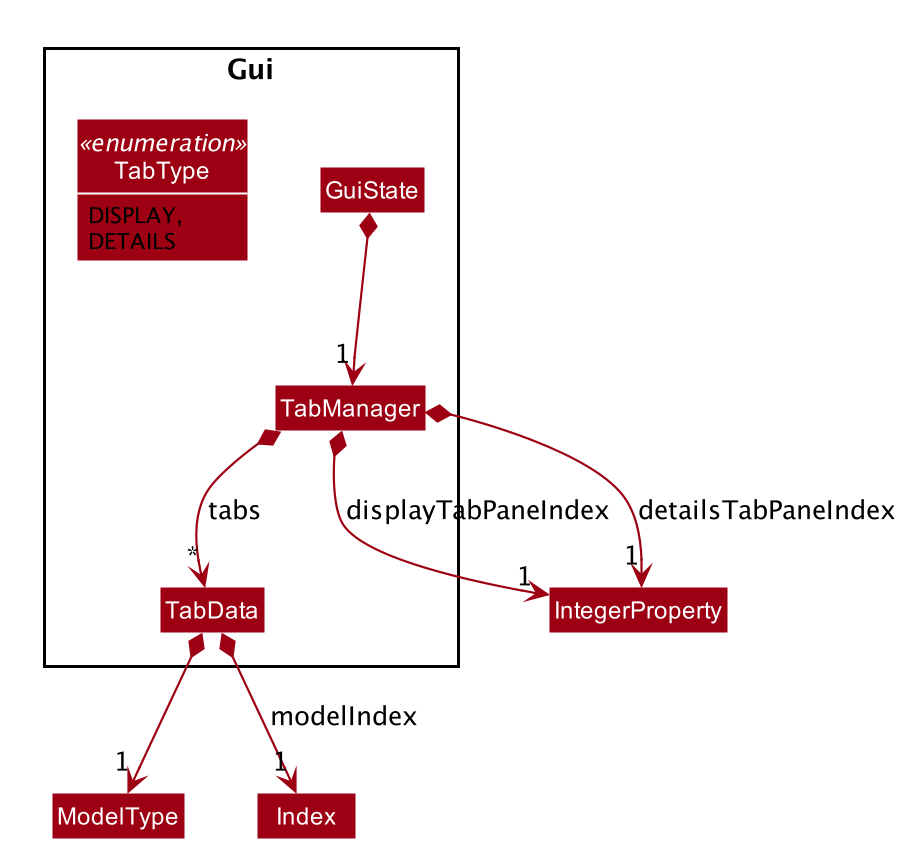
GuiState
classThe following Activity diagram illustrates the series of actions that occur when the user opens a new tab:
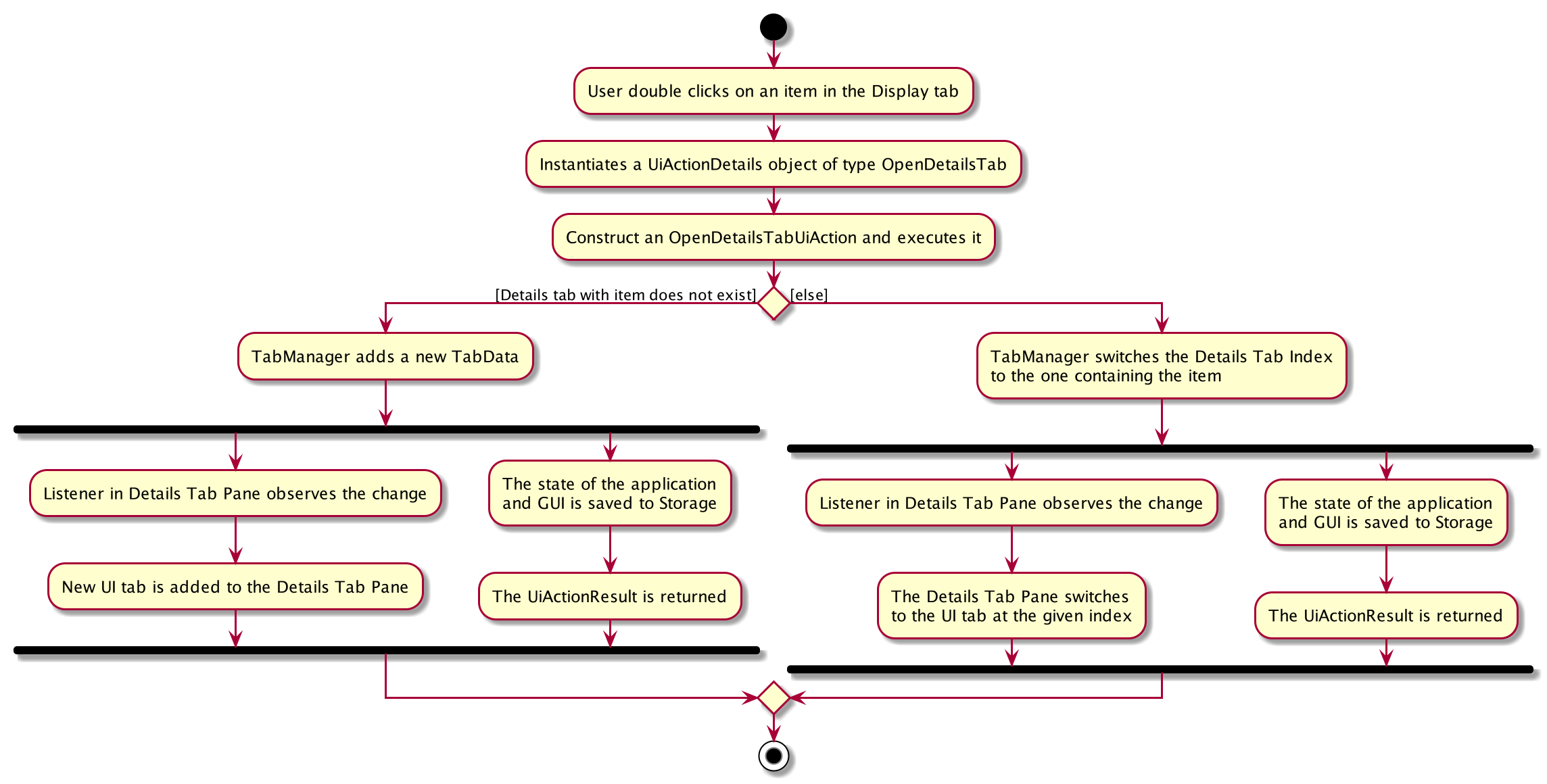
In AlgoBase, the state of the GUI is also saved to Storage after every action. This is so that when the user closes the application and opens it again later, the state is stored. The Sequence diagram below also shows how the GuiState is saved to Storage:
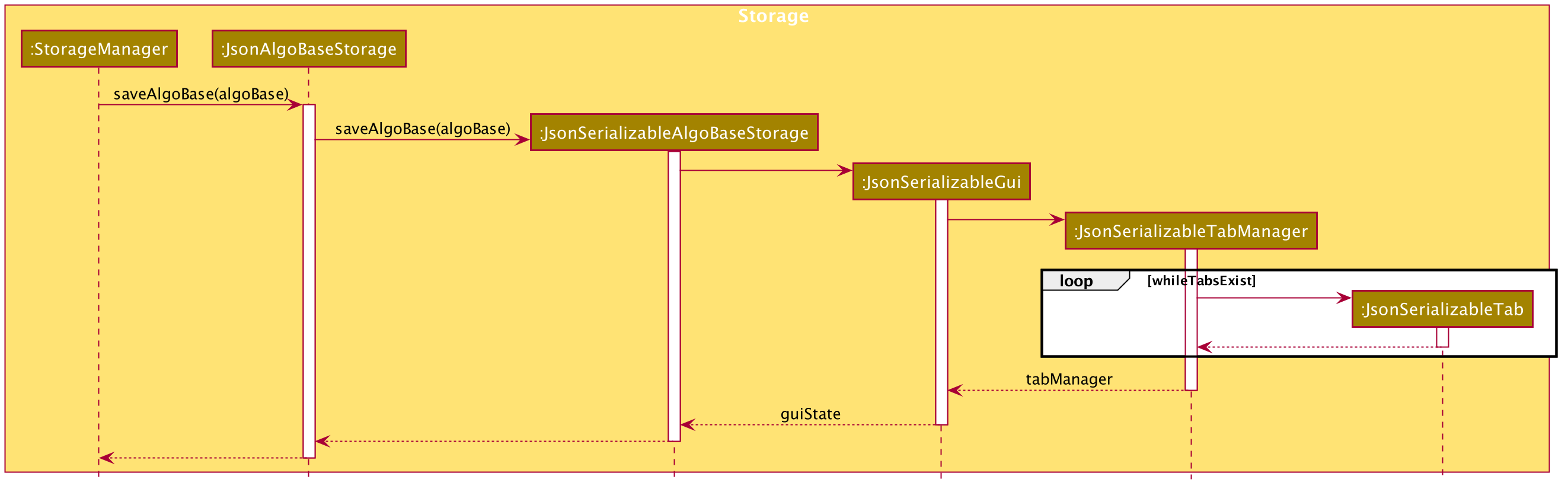
The StorageManager
saves the modified GuiState
as a new JSON
file.
This is done with the help of the JsonSerializableGui
, JsonSerializableTabManager
and JsonSerializableTab
classes that are wrappers for the GuiState
, TabManager
and TabData
classes.
These wrapper classes can be converted into JSON
format for storage without any data loss.
Design Considerations
Aspect | Alternative 1 (Current Choice) | Alternative 2 |
---|---|---|
How to implement Commands and UI Actions in the same application |
Handle Commands and UI Actions separately. Pros: Higher modularity. Allows separation the different architectures as well (Synchronous for Commands & Event-Driven for UI Actions) Cons: Multiple Logic managers (LogicManager and UiLogicManager) |
Handle Commands and UI Actions together. Pros: Less code and higher reusability. Cons: Higher coupling and less cohesion. |
How to handle different kinds of UI Actions |
Using a command structure with a central parser and many smaller parsers. Pros: Higher extensibility, easier to add new UI Actions Cons: Have to write more code to achieve the same functionality. |
Handling each UI action individually. Pros: Can write less code to achieve the same functionality. Cons: Lower extensibility, harder to add new UI Actions |